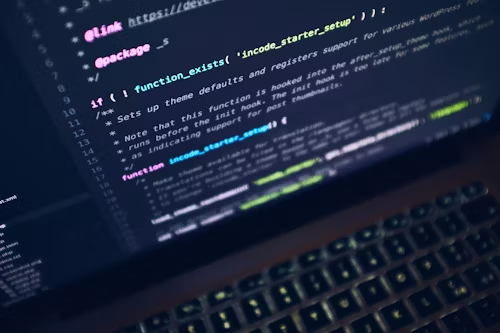
One intends to apply the two-pointer strategy. But the array has to be sorted if one is utilizing the two- pointer approach. Once the array is sorted, we can apply this method retaining one pointer at the beginning (left) and another at the end (right) of the array. Check the sum of the components at these two pointers next as well:
- We have located the pair if the total comes equal to the target.
- Move the left cursor to the right to raise the sum should it be less than the aim.
- Move the right pointer to the left to lower the amount should it be more than the aim.
C++
#include <bits/stdc++.h>
using namespace std;
// Function to check whether any pair exists
// whose sum is equal to the given target value
bool twoSum(vector<int> &arr, int target){
// Sort the array
sort(arr.begin(), arr.end());
int left = 0, right = arr.size() - 1;
// Iterate while left pointer is less than right
while (left < right){
int sum = arr[left] + arr[right];
// Check if the sum matches the target
if (sum == target)
return true;
else if (sum < target)
left++; // Move left pointer to the right
else
right--; // Move right pointer to the left
}
// If no pair is found
return false;
}
int main(){
vector<int> arr = {0, -1, 2, -3, 1};
int target = -2;
// Call the twoSum function and print the result
if (twoSum(arr, target))
cout << "true";
else
cout << "false";
return 0;
}
C
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
// Comparison function for qsort
int compare(const void *a, const void *b){
return (*(int *)a - *(int *)b);
}
// Function to check whether any pair exists
// whose sum is equal to the given target value
bool twoSum(int arr[], int n, int target){
// Sort the array
qsort(arr, n, sizeof(int), compare);
int left = 0, right = n - 1;
// Iterate while left pointer is less than right
while (left < right){
int sum = arr[left] + arr[right];
// Check if the sum matches the target
if (sum == target)
return true;
else if (sum < target)
left++; // Move left pointer to the right
else
right--; // Move right pointer to the left
}
// If no pair is found
return false;
}
int main(){
int arr[] = {0, -1, 2, -3, 1};
int target = -2;
int n = sizeof(arr) / sizeof(arr[0]);
// Call the twoSum function and print the result
if (twoSum(arr, n, target))
printf("true\n");
else
printf("false\n");
return 0;
}
Java
import java.util.Arrays;
class GfG {
// Function to check whether any pair exists
// whose sum is equal to the given target value
static boolean twoSum(int[] arr, int target){
// Sort the array
Arrays.sort(arr);
int left = 0, right = arr.length - 1;
// Iterate while left pointer is less than right
while (left < right) {
int sum = arr[left] + arr[right];
// Check if the sum matches the target
if (sum == target)
return true;
else if (sum < target)
left++; // Move left pointer to the right
else
right--; // Move right pointer to the left
}
// If no pair is found
return false;
}
public static void main(String[] args){
int[] arr = { 0, -1, 2, -3, 1 };
int target = -2;
// Call the twoSum function and print the result
if (twoSum(arr, target)) {
System.out.println("true");
}
else {
System.out.println("false");
}
}
}
Python
# Function to check whether any pair exists
# whose sum is equal to the given target value
def two_sum(arr, target):
# Sort the array
arr.sort()
left, right = 0, len(arr) - 1
# Iterate while left pointer is less than right
while left < right:
sum = arr[left] + arr[right]
# Check if the sum matches the target
if sum == target:
return True
elif sum < target:
left += 1 # Move left pointer to the right
else:
right -= 1 # Move right pointer to the left
# If no pair is found
return False
arr = [0, -1, 2, -3, 1]
target = -2
# Call the two_sum function and print the result
if two_sum(arr, target):
print("true")
else:
print("false")
Output
1
Time Complexity: O(n*log(n)), for sorting the array
Auxiliary Space: O(1)