One of the basic graphs traversal techniques is Breadth First Search (BFS). It starts with a node and first moves all around it. Their neighboring are walked once all adjacent have been visited. Unlike DFS, this visits closest vertices before others in a different sense. We mostly move vertically level by level. Many well-known graph algorithms, including Prim’s algorithm, Kahn’s Algorithm, and Dijkstra’s shortest path, depend on BFS. BFS itself finds shortest path in an unweighted graph, detects cycle in both directed and undirected graphs, and many more issues.
Starting from a given source, the program investigates all reachable vertices from the supplied source. It bears resemblance to a tree’s Breadth-First Traversal. Like tree, we start with the supplied source (in tree, we start with root) and move vertices level by level employing a queue data structure. The sole catch here is that graphs could include cycles, unlike trees, thus we might find the same node once again. We employ a boolean visited array to prevent repeatedly processing a node.
Enqueue the specified source vertex and mark it as visited initially.
- Exploration: Dequeue a node from the queue and visit it (e.g., print its value), even though the queue isn’t empty.
- Enqueue every unvisited neighbor of the dequeued node in turn.
- Noted the neighbor as visited.
- Termination: Continue step 2 till the line runs empty.
- Beginning with the starting node, this technique guarantees that every node in the graph is visited in a breadth-first sequence.
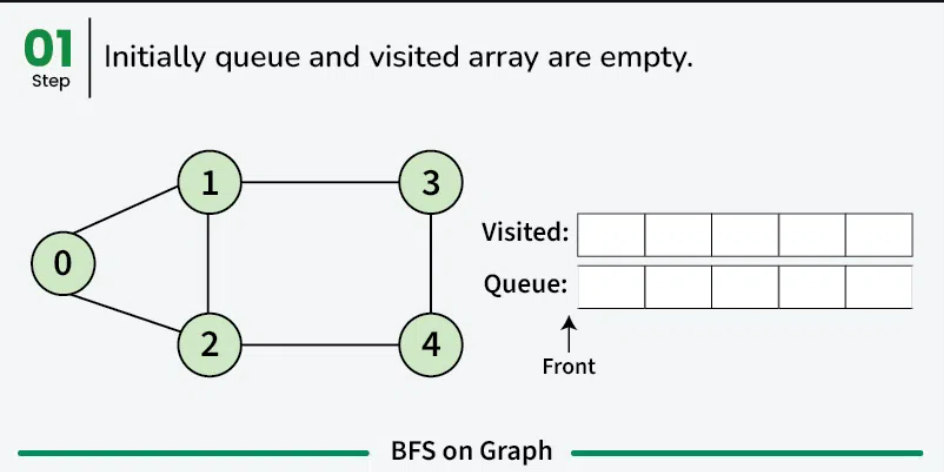
BFS of the whole Graph maybe disconnected
In case of a disconnected graph, the aforementioned code prints just those vertices that are reachable from the source, thereby depending on a source it accepts as input. Now let us discuss the graph maybe disconnected and the method that displays all vertices without any source.
The concept is straightforward: instead of requesting BFS for a single vertex, we call the above designed BFS one by one for all non-visited vertices.
Breadth-First Search (BFS) Algorithm: A Complexity Study
Time Complexity of the BFS Algorithm: O(V + E)
BFS investigates every graph edge and vertex. Under worst circumstances, it visits every edge and vertex once. BFS’s time complexity is thus O(V + E), where V and E are the provided graph’s vertex and edge counts respectively.
BFS Algorithm Auxiliary Space: O(V)
To mark the vertices that must be visited, BFS employs a queue. The queue may, in the worst situation include every vertex in the graph. BFS has therefore O(V) as its space complexity.
BFS uses in graphs:
In computer science and graph theory, BFS finds uses including:
- In an unweighted network, BFS allows one to determine the shortest path between two nodes. Reconstructed shortest path can be obtained by tracking parent of every node during traversal.
- Graph cycles can be found with BFS by means of which Should a node be visited twice during the traverse, a cycle exists.
- BFS allows one to find connected components in a graph. Every connected component is an assembly of nodes accessible from one another.
- On a directed acyclic graph (DAG), BFS can applied for topological sorting. Topological sorting sets the nodes in a linear sequence whereby u appears before v in the sequence for any edge (u, v).
- Level Order Traversal of Binary Trees: A binary tree can level ordered using BFS. This traversal visits every node at the same level then moves to the next level.
Data packet routing in network protocols benefits from BFS’s ability to determine the shortest path between two nodes in a network.
Breadth First Search (BFS) for a Graph FAQs
First question: What is BFS and how operates?
BFS is a graph traversal method whereby a graph methodically explored visiting all the vertices at a particular level then proceeding to the following level. Beginning at a starting vertex, it enqueues it into a queue and notes it as visited. It then visits a vertex from the queue, dequeues all of her unvisited neighbors into the queue, This process keeps on till the queue runs empty.
Second question: For what uses BFS finds appropriate?
BFS finds the shortest path in an unweighted graph, detects cycles in a graph, topologically sorts a directed acyclic graph (DAG), identifies related components in a graph, and solves mazes and Sudoku puzzles among other things.
What is BFS’s temporal complexity in question three?
BFS’s temporal complexity is O(V + E), where V is the graph’s vertex count and E its edge count.
Fourth question: How complexly space BFS uses?
BFS employs a queue to monitor the vertices that must be visited, so its space complexity is O(V).
The benefits of BFS use include question five.
For an unweighted graph, BFS is straightforward to apply and effective for determining the shortest path. It ensures furthermore that every vertex in the graph gets visited.