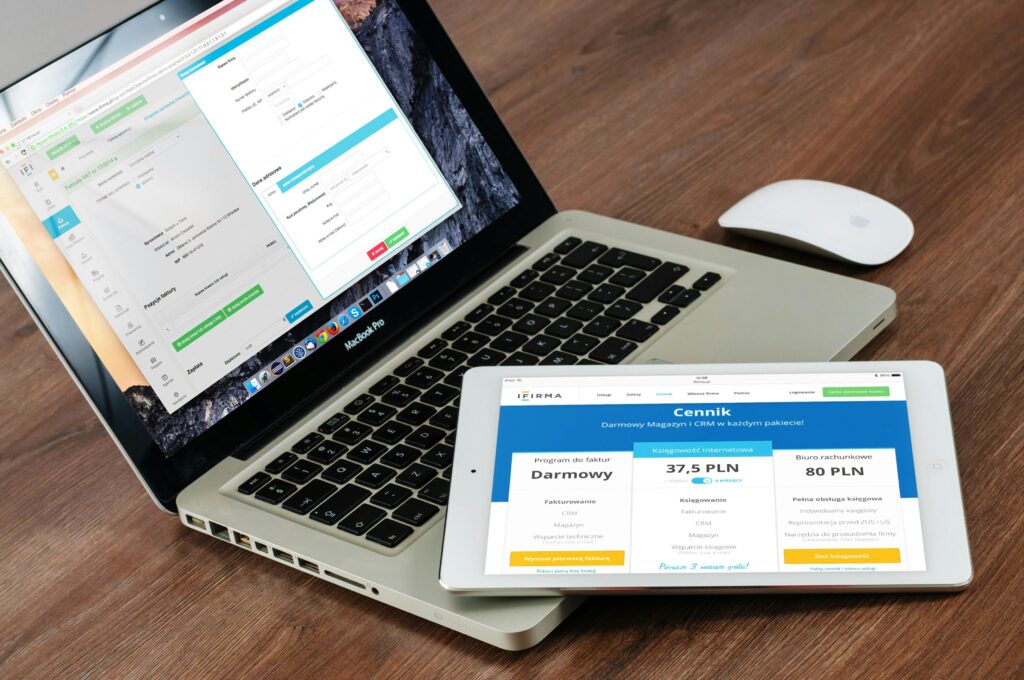
Examples:
Input : 9
Output : IX
Input : 40
Output : XL
Input : 1904
Output : MCMIV
Following is the list of Roman symbols which include subtractive cases also:
SYMBOL VALUE
I 1
IV 4
V 5
IX 9
X 10
XL 40
L 50
XC 90
C 100
CD 400
D 500
CM 900
M 1000
The plan is to separately convert the tens, hundreds, thousands of sites of the specified number. There is no matching Roman numeral symbol if the digit is zero. Because these digits use subtractive notation, the conversion of digits 4’s and 9’s is somewhat different from other digits.
Method for Roman numeral conversion from decimal number
Comparing provided number with base values in the sequence 1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1, The first base value—largest base value—will be either exactly less or equal to the specified integer.Divide the number by its highest base value; the corresponding base symbol will be repeated quotient times; the residual will then be the number for next divisions and repetitions. The cycle will be continued until the count runs zero.
Example to demonstrate above algorithm:
Convert 3549 to its Roman Numerals
Output:
MMMDXLIX
Explanation:
First step:
First number = 3549
Since 3549 > 1000; first base value will be 1000 initially.
Get 3549/1000 divided. Remainder =549; quotient = 3. Three times will be the equivalent sign M repeated.
We list the Result value in the second list.
Now remainder is not equal to zero hence we call the method once more.
Two steps
Number now is 549; maximum base value will be 500; 1000 > 549 >= 500.
Split 549/500. Remainder: 49; quotient: 1. Once, the matching symbol D will be repeated to stop the loop.
We list the Result value in the second list.
Now remainder is not equal to zero hence we call the method once more.
The third step
Number now is 49; maximum base value is 40; 50 > 49 >= 40.
Sort 49/40. Quotient is one; remainder is nine. Once, the matching symbol XL will be repeated to stop the loop.
We list the Result value in the second list.
Now remainder is not equal to zero hence we call the method once more.
Step Four
Now, number is 9.
List l contains number 9; so, we straight get the value from dictionary dict and set Remainder=0 and finish the loop.
Remainder= 0. We shall not call the method once more since the related symbol IX will be repeated once and the remaining value is 0.
Step five
At last, we align with the second list values.
The result came MMMDXLIX.
The above method is applied following here:
C++
// C++ Program to convert decimal number to
// roman numerals
#include <bits/stdc++.h>
using namespace std;
// Function to convert decimal to Roman Numerals
int printRoman(int number)
{
int num[] = {1,4,5,9,10,40,50,90,100,400,500,900,1000};
string sym[] = {"I","IV","V","IX","X","XL","L","XC","C","CD","D","CM","M"};
int i=12;
while(number>0)
{
int div = number/num[i];
number = number%num[i];
while(div--)
{
cout<<sym[i];
}
i--;
}
}
//Driver program
int main()
{
int number = 3549;
printRoman(number);
return 0;
}
Java
// Java Program to convert decimal number to
// roman numerals
class GFG {
// To add corresponding base symbols in the array
// to handle cases that follow subtractive notation.
// Base symbols are added index 'i'.
static int sub_digit(char num1, char num2, int i, char[] c) {
c[i++] = num1;
c[i++] = num2;
return i;
}
// To add symbol 'ch' n times after index i in c[]
static int digit(char ch, int n, int i, char[] c) {
for (int j = 0; j < n; j++) {
c[i++] = ch;
}
return i;
}
// Function to convert decimal to Roman Numerals
static void printRoman(int number) {
char c[] = new char[10001];
int i = 0;
// If number entered is not valid
if (number <= 0) {
System.out.printf("Invalid number");
return;
}
// TO convert decimal number to roman numerals
while (number != 0) {
// If base value of number is greater than 1000
if (number >= 1000) {
// Add 'M' number/1000 times after index i
i = digit('M', number / 1000, i, c);
number = number % 1000;
} // If base value of number is greater than or
// equal to 500
else if (number >= 500) {
// To add base symbol to the character array
if (number < 900) {
// Add 'D' number/1000 times after index i
i = digit('D', number / 500, i, c);
number = number % 500;
} // To handle subtractive notation in case of number
// having digit as 9 and adding corresponding base
// symbol
else {
// Add C and M after index i/.
i = sub_digit('C', 'M', i, c);
number = number % 100;
}
} // If base value of number is greater than or equal to 100
else if (number >= 100) {
// To add base symbol to the character array
if (number < 400) {
i = digit('C', number / 100, i, c);
number = number % 100;
} // To handle subtractive notation in case of number
// having digit as 4 and adding corresponding base
// symbol
else {
i = sub_digit('C', 'D', i, c);
number = number % 100;
}
} // If base value of number is greater than or equal to 50
else if (number >= 50) {
// To add base symbol to the character array
if (number < 90) {
i = digit('L', number / 50, i, c);
number = number % 50;
} // To handle subtractive notation in case of number
// having digit as 9 and adding corresponding base
// symbol
else {
i = sub_digit('X', 'C', i, c);
number = number % 10;
}
} // If base value of number is greater than or equal to 10
else if (number >= 10) {
// To add base symbol to the character array
if (number < 40) {
i = digit('X', number / 10, i, c);
number = number % 10;
} // To handle subtractive notation in case of
// number having digit as 4 and adding
// corresponding base symbol
else {
i = sub_digit('X', 'L', i, c);
number = number % 10;
}
} // If base value of number is greater than or equal to 5
else if (number >= 5) {
if (number < 9) {
i = digit('V', number / 5, i, c);
number = number % 5;
} // To handle subtractive notation in case of number
// having digit as 9 and adding corresponding base
// symbol
else {
i = sub_digit('I', 'X', i, c);
number = 0;
}
} // If base value of number is greater than or equal to 1
else if (number >= 1) {
if (number < 4) {
i = digit('I', number, i, c);
number = 0;
} // To handle subtractive notation in case of
// number having digit as 4 and adding corresponding
// base symbol
else {
i = sub_digit('I', 'V', i, c);
number = 0;
}
}
}
// Printing equivalent Roman Numeral
System.out.printf("Roman numeral is: ");
for (int j = 0; j < i; j++) {
System.out.printf("%c", c[j]);
}
}
//Driver program
public static void main(String[] args) {
int number = 3549;
printRoman(number);
}
}
// This code is contributed by PrinciRaj1992
Python
# Python3 program to convert
# decimal number to roman numerals
ls=[1000,900,500,400,100,90,50,40,10,9,5,4,1]
dict={1:"I",4:"IV",5:"V",9:"IX",10:"X",40:"XL",50:"L",90:"XC",100:"C",400:"CD",500:"D",900:"CM",1000:"M"}
ls2=[]
# Function to convert decimal to Roman Numerals
def func(no,res):
for i in range(0,len(ls)):
if no in ls:
res=dict[no]
rem=0
break
if ls[i]<no:
quo=no//ls[i]
rem=no%ls[i]
res=res+dict[ls[i]]*quo
break
ls2.append(res)
if rem==0:
pass
else:
func(rem,"")
# Driver code
if __name__ == "__main__":
func(3549, "")
print("".join(ls2))
# This code is contributed by
# VIKAS CHOUDHARY(vikaschoudhary344)
C#
<script>
// JavaScript Program to convert decimal number to
// roman numerals
// Function to convert decimal to Roman Numerals
function printRoman(number)
{
let num = [1,4,5,9,10,40,50,90,100,400,500,900,1000];
let sym = ["I","IV","V","IX","X","XL","L","XC","C","CD","D","CM","M"];
let i=12;
while(number>0)
{
let div = Math.floor(number/num[i]);
number = number%num[i];
while(div--)
{
document.write(sym[i]);
}
i--;
}
}
//Driver program
let number = 3549;
printRoman(number);
//This code is contributed by Manoj
</script>
Output
MMMDXLIX
Time Complexity: O(N), where N is the length of ans string that stores the conversion.
Auxiliary Space: O(N)