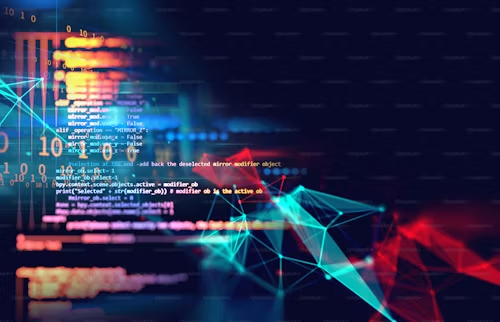
Overview
Floyd’s cycle detection approach is a pointer algorithm that traverses the sequence at varying speeds using just two points. The application of this approach in Linked Lists and its results are described in the paper.
Finding out if the linked list has a cycle or not is the goal. The head node’s two pointers are kept first. You move one of the pointers by one step and the other by two steps at each iteration. The tortoise and the hare are your two pointers. One of the two scenarios will eventually occur:
Hare will arrive at the linked list’s tail (null), indicating that it is cycle-free.
When a tortoise meets a hare, a cycle is in place.
The following is the most logical way to interpret this:
The hare walks two nodes and the tortoise walks one node at each stage of the algorithm. In actuality, the hare approaches after the tortoise has been gone for one unit of distance. In actuality, the hare moves one step and the tortoise remains still with each step.
The Hare-Tortoise algorithm, also known as Floyd’s cycle finding algorithm, is a pointer algorithm that employs just two pointers and traverses the sequence at varying speeds. A loop in a linked list can be located using this approach. Two pointers are used, one of which moves twice as quickly as the other. The two are referred to as the slow pointer and the quick pointer, respectively.
One of these things will happen as you navigate the linked list:
There is no loop in the linked list if the Fast pointer reaches the end (NULL).
There is a loop in the linked list because the fast pointer eventually catches the slow pointer again.
Floyd’s Cycle Finding Algorithm:
The two pointers, slow and fast, supposed to begin at the top of the linked list.
- One step at a time, the sluggish cursor will navigate the list.
- Two steps at a time taken by the rapid pointer.
- In the event of a cycle, the fast pointer will eventually overtake the slow pointer because it is traveling more quickly.
- The fast pointer will reach the end of the list (becoming NULL) if there is no cycle.
- A cycle or loop is created when the slow and fast points come together.
What is the operation of Floyd’s Algorithm?
Two pointers—slow and fast—are started from the head of the linked list by the algorithm. One node at a time, we progress slowly, and two nodes at a time, we move quickly. They will undoubtedly meet if there is a loop.
The following facts make this strategy effective:
The fast pointer needs to be inside the loop when the slow pointer enters it.
We may observe that the distance between slow and fast pointers increases by one with each iteration if we look at how they move.
The distance between the two pointers grows after each repetition in which the slow pointer advances one step and the fast pointer advances two. The distance between the slow and fast pointers increases k+1 after one iteration if the slow pointer is initially k from a specific point in the cycle. This distance becomes k+2 after two iterations, and so forth. This distance will eventually match the cycle length n as they keep moving within the cycle. Since both points are traveling within the same cycle and the distance is now encircling the cycle, they will collide.
Please see the page “How does Floyd’s Algorithm work?” for further information on the algorithm’s operation and proof.
Locate a Cycle’s Beginning Node in a Linked List
Use the following procedures to determine a cycle’s beginning node in a linked list:
The meeting point (assuming a cycle exists) where the slow and fast pointers intersect inside the cycle can be found using the procedure above.
Reset one pointer (slow) to the list’s head after identifying the cycle. At the meeting spot, keep the other pointer (quick).
One step at a time, move both pointers. The cycle begins at the node where they reunite.
Benefits: It uses extremely little memory because it does not require additional space (such as a hash table) to record visited nodes.
The middle element of a linked list can also be found using an adaptation of this approach.

Learn about the Two Pointers method.
Let me start by introducing a challenging technique from the field of problem-solving. Determine the middle node in a linked list. List data structures should known to you. Otherwise, kindly learn it before proceeding.
Our circles are therefore nodes of a linked list based on the images above.
The middle ones on the list are the red ones. Both nodes (3) and (4) can be regarded as middle nodes if the list is even in length. However, let’s assume that only node (4) regarded as a middle. You have to locate the red circle (a midpoint) and bring it back. Please take note that you are unaware of the list’s length (number of nodes), foxy fella. The solution’s complexity should be as little as feasible.
Counting every node by iterating them would be the most obvious solution. After that, go over it again, stopping at half of the count. Lovely! You are currently halfway through the list. The issue has resolved! However, is it truly a quick fix? You see, you have to go through your list nearly twice. What if I told you that there is only one traversal that can solve it?
Two Pointers is the name of the trick. A second pointer must introduced. Your default iterator, which advances one step at a time, is the first one. Your slow tortoise is it. Your hare is the second one. It takes two steps all at once.