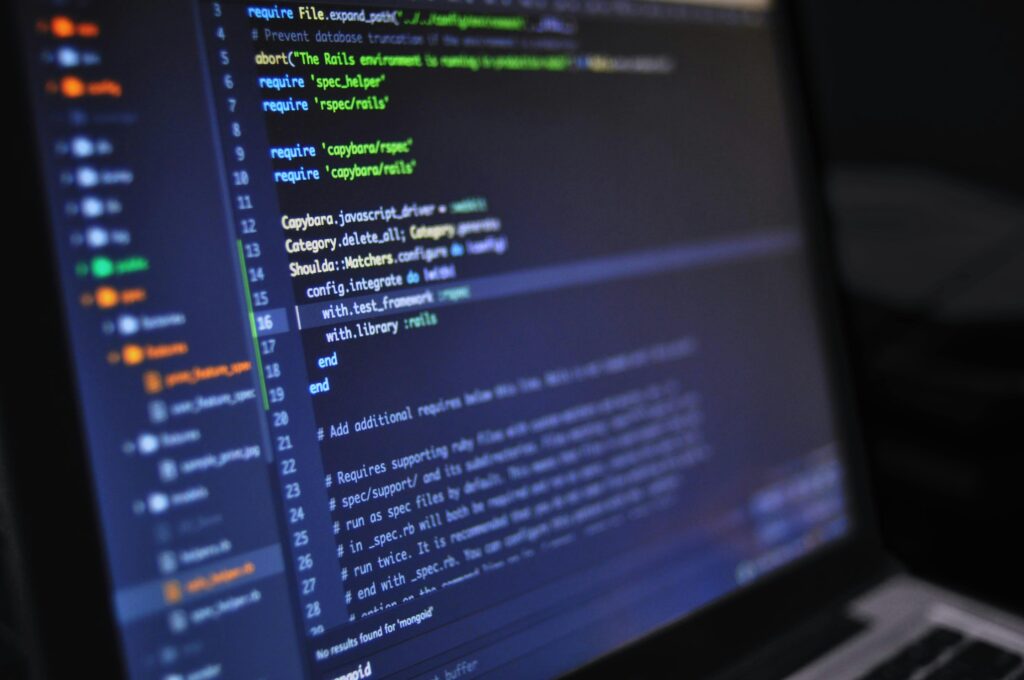
Roman symbol values may be kept using an unordered map. We must thus cycle through the string and for every symbol see whether the present value is less than the next value in order to solve the puzzle. If so, add the outcome of subtracting the current value from the next value to the total. Add the current value to the total otherwise.
C++
#include <bits/stdc++.h>
using namespace std;
int romanToDecimal(string& str) {
unordered_map<char, int> romanMap = {
{'I', 1}, {'V', 5}, {'X', 10},
{'L', 50}, {'C', 100}, {'D', 500}, {'M', 1000}
};
// Initialize result
int sum = 0;
for (int i = 0; i < str.length(); i++) {
// If the current value is less than the next value, subtract current from next and add to sum
if (i + 1 < str.length() && romanMap[str[i]] < romanMap[str[i + 1]]) {
sum += romanMap[str[i + 1]] - romanMap[str[i]];
// Skip the next symbol
i++;
} else {
// Otherwise, add the current value to sum
sum += romanMap[str[i]];
}
}
return sum;
}
// Driver code
int main() {
string str = "IX";
cout << romanToDecimal(str) << endl;
return 0;
}
Java
import java.util.HashMap;
public class RomanToDecimal {
public static int romanToDecimal(String str)
{
HashMap<Character, Integer> romanMap
= new HashMap<>();
romanMap.put('I', 1);
romanMap.put('V', 5);
romanMap.put('X', 10);
romanMap.put('L', 50);
romanMap.put('C', 100);
romanMap.put('D', 500);
romanMap.put('M', 1000);
int sum = 0;
for (int i = 0; i < str.length(); i++) {
// If the current value is less than the next
// value, subtract current from next and add to
// sum
if (i + 1 < str.length()
&& romanMap.get(str.charAt(i))
< romanMap.get(str.charAt(i + 1))) {
sum += romanMap.get(str.charAt(i + 1))
- romanMap.get(str.charAt(i));
// Skip the next symbol
i++;
}
else {
// Otherwise, add the current value to sum
sum += romanMap.get(str.charAt(i));
}
}
return sum;
}
public static void main(String[] args)
{
String str = "IX";
System.out.println(romanToDecimal(str));
}
}
Python
def romanToDecimal(s: str) -> int:
roman_map = {
'I': 1, 'V': 5, 'X': 10,
'L': 50, 'C': 100, 'D': 500, 'M': 1000
}
# Initialize result
sum = 0
i = 0
while i < len(s):
# If the current value is less than the next
# value, subtract current from next and add to sum
if i + 1 < len(s) and roman_map[s[i]] < roman_map[s[i + 1]]:
sum += roman_map[s[i + 1]] - roman_map[s[i]]
# Skip the next symbol
i += 2
else:
# Otherwise, add the current value to sum
sum += roman_map[s[i]]
i += 1
return sum
# Driver code
if __name__ == "__main__":
s = "IX"
print(romanToDecimal(s))
Js
function romanToDecimal(str) {
const romanMap = {
'I': 1,
'V': 5,
'X': 10,
'L': 50,
'C': 100,
'D': 500,
'M': 1000
};
let sum = 0;
for (let i = 0; i < str.length; i++) {
// If the current value is less than the next value,
// subtract current from next and add to sum
if (i + 1 < str.length && romanMap[str[i]] < romanMap[str[i + 1]]) {
sum += romanMap[str[i + 1]] - romanMap[str[i]];
// Skip the next symbol
i++;
} else {
// Otherwise, add the current value to sum
sum += romanMap[str[i]];
}
}
return sum;
}
console.log(romanToDecimal("IX")); // Output: 9
Output
9
Time Complexity: O(N), As we have to iterate the string only once.
Auxiliary Space: O(1), As we are using a map of constant size, so the space complexity becomes O(1)